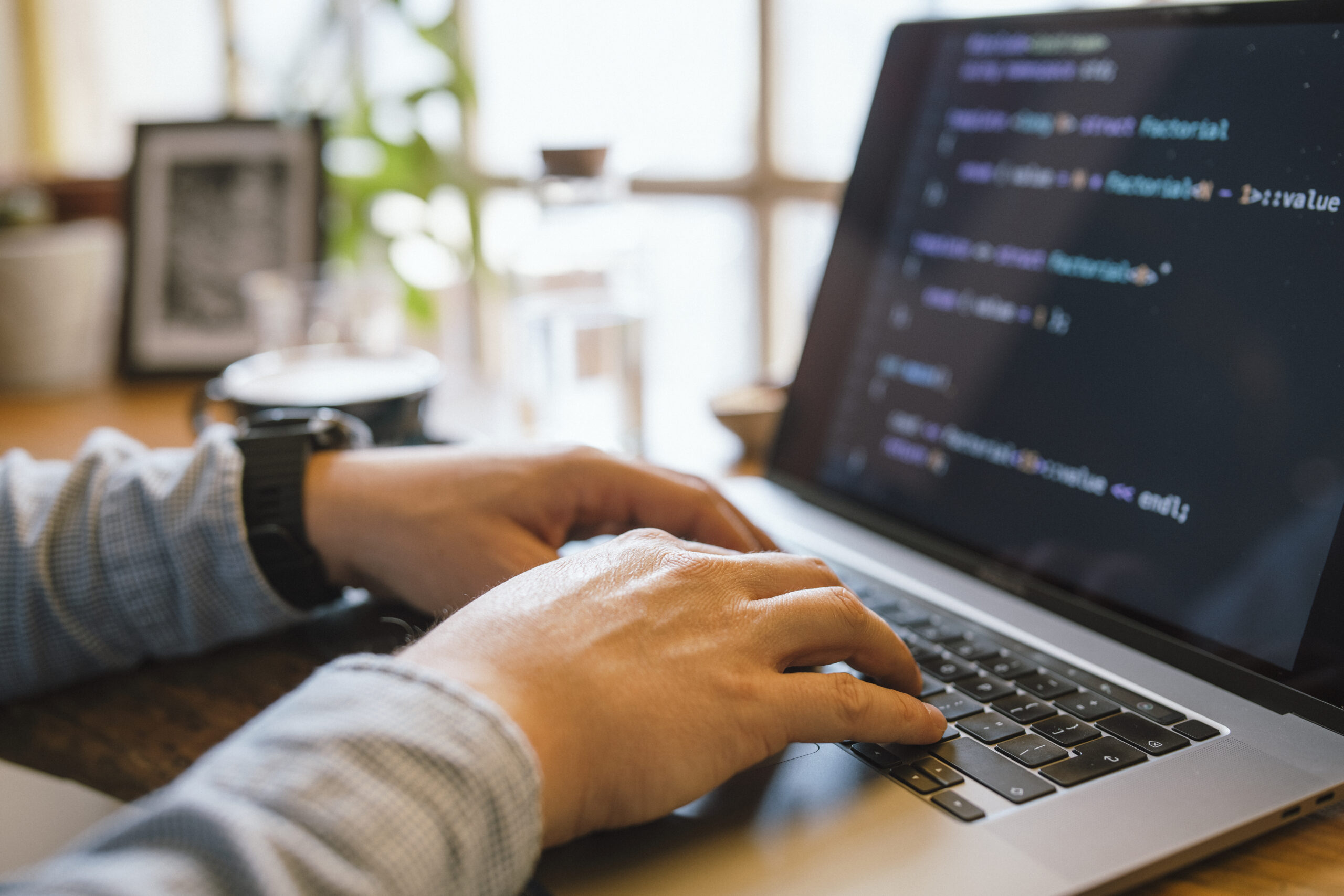
Debugging is Just about the most critical — however usually forgotten — competencies in a developer’s toolkit. It isn't nearly fixing broken code; it’s about comprehension how and why factors go Erroneous, and Discovering to Imagine methodically to unravel complications competently. Whether you're a starter or a seasoned developer, sharpening your debugging skills can help you save several hours of irritation and radically help your efficiency. Here's various approaches that can help developers degree up their debugging sport by me, Gustavo Woltmann.
Master Your Applications
On the list of fastest techniques developers can elevate their debugging skills is by mastering the tools they use every day. While crafting code is one Element of enhancement, figuring out the best way to interact with it effectively all through execution is equally essential. Fashionable progress environments appear equipped with impressive debugging capabilities — but numerous builders only scratch the floor of what these resources can perform.
Acquire, as an example, an Built-in Growth Surroundings (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment allow you to established breakpoints, inspect the worth of variables at runtime, phase through code line by line, and in many cases modify code around the fly. When made use of appropriately, they Permit you to observe accurately how your code behaves in the course of execution, which is priceless for monitoring down elusive bugs.
Browser developer resources, for instance Chrome DevTools, are indispensable for front-conclude builders. They let you inspect the DOM, observe network requests, look at true-time functionality metrics, and debug JavaScript in the browser. Mastering the console, sources, and network tabs can convert irritating UI difficulties into manageable duties.
For backend or process-level developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB provide deep Management around operating procedures and memory administration. Studying these equipment could possibly have a steeper learning curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn out to be relaxed with Model Command methods like Git to comprehend code heritage, obtain the precise minute bugs were being introduced, and isolate problematic modifications.
In the end, mastering your equipment suggests likely past default options and shortcuts — it’s about establishing an personal familiarity with your progress ecosystem so that when problems arise, you’re not misplaced at midnight. The better you understand your equipment, the more time you'll be able to devote solving the actual problem rather than fumbling through the procedure.
Reproduce the condition
One of the more significant — and infrequently forgotten — methods in powerful debugging is reproducing the trouble. Prior to jumping into your code or building guesses, developers require to create a dependable natural environment or circumstance in which the bug reliably appears. Without the need of reproducibility, repairing a bug gets to be a game of possibility, usually bringing about wasted time and fragile code changes.
The initial step in reproducing a difficulty is gathering just as much context as you possibly can. Ask issues like: What actions triggered The problem? Which atmosphere was it in — enhancement, staging, or creation? Are there any logs, screenshots, or error messages? The greater detail you may have, the less difficult it will become to isolate the exact ailments below which the bug takes place.
After you’ve gathered ample information, endeavor to recreate the issue in your neighborhood atmosphere. This might mean inputting the exact same information, simulating very similar user interactions, or mimicking technique states. If The difficulty appears intermittently, look at writing automated assessments that replicate the sting circumstances or point out transitions involved. These exams not simply assist expose the challenge but will also avoid regressions Sooner or later.
Sometimes, The problem can be environment-certain — it would materialize only on particular working devices, browsers, or less than specific configurations. Employing instruments like Digital machines, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these bugs.
Reproducing the condition isn’t just a stage — it’s a frame of mind. It involves tolerance, observation, and a methodical technique. But when you can persistently recreate the bug, you happen to be by now midway to correcting it. Which has a reproducible state of affairs, you can use your debugging tools much more successfully, check prospective fixes securely, and talk a lot more Obviously along with your crew or consumers. It turns an summary criticism right into a concrete problem — and that’s in which developers thrive.
Browse and Have an understanding of the Mistake Messages
Mistake messages are sometimes the most useful clues a developer has when anything goes Improper. As opposed to viewing them as frustrating interruptions, builders really should understand to deal with error messages as immediate communications with the technique. They usually tell you just what took place, wherever it occurred, and occasionally even why it transpired — if you understand how to interpret them.
Commence by studying the information meticulously and in comprehensive. Quite a few developers, specially when below time pressure, look at the initial line and immediately start out generating assumptions. But deeper from the error stack or logs may perhaps lie the real root cause. Don’t just duplicate and paste error messages into search engines — read through and comprehend them initially.
Break the mistake down into components. Can it be a syntax error, a runtime exception, or maybe a logic error? Does it point to a certain file and line number? What module or operate brought on it? These queries can guideline your investigation and level you towards the responsible code.
It’s also valuable to understand the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally adhere to predictable designs, and Finding out to acknowledge these can significantly hasten your debugging process.
Some mistakes are imprecise or generic, As well as in These instances, it’s essential to look at the context wherein the error transpired. Test related log entries, input values, and recent improvements in the codebase.
Don’t forget about compiler or linter warnings both. These normally precede bigger troubles and supply hints about opportunity bugs.
Finally, error messages usually are not your enemies—they’re your guides. Mastering to interpret them correctly turns chaos into clarity, assisting you pinpoint concerns more rapidly, cut down debugging time, and turn into a more efficient and assured developer.
Use Logging Properly
Logging is The most highly effective applications inside of a developer’s debugging toolkit. When used successfully, it provides genuine-time insights into how an application behaves, assisting you comprehend what’s taking place under the hood with no need to pause execution or stage with the code line by line.
An excellent logging method begins with realizing what to log and at what degree. Typical logging ranges include things like DEBUG, Facts, Alert, ERROR, and FATAL. Use DEBUG for comprehensive diagnostic information during development, Facts for normal gatherings (like profitable commence-ups), get more info WARN for possible issues that don’t crack the appliance, ERROR for precise challenges, and Deadly when the procedure can’t go on.
Prevent flooding your logs with extreme or irrelevant information. Too much logging can obscure significant messages and slow down your process. Target crucial events, condition adjustments, enter/output values, and significant choice details within your code.
Format your log messages Plainly and regularly. Involve context, for example timestamps, request IDs, and performance names, so it’s easier to trace troubles in distributed programs or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re Primarily useful in output environments in which stepping as a result of code isn’t achievable.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. That has a well-imagined-out logging solution, you'll be able to lessen the time it takes to spot difficulties, gain deeper visibility into your apps, and Increase the overall maintainability and dependability of your respective code.
Think Like a Detective
Debugging is not only a complex endeavor—it's a type of investigation. To proficiently detect and repair bugs, developers have to tactic the procedure like a detective solving a thriller. This way of thinking helps break down intricate difficulties into workable parts and stick to clues logically to uncover the basis bring about.
Start by gathering evidence. Look at the signs of the situation: mistake messages, incorrect output, or general performance issues. Much like a detective surveys a crime scene, gather as much pertinent data as it is possible to devoid of leaping to conclusions. Use logs, test scenarios, and person experiences to piece alongside one another a transparent photograph of what’s going on.
Next, form hypotheses. Inquire your self: What may be triggering this conduct? Have any adjustments not too long ago been manufactured on the codebase? Has this situation occurred prior to below similar circumstances? The intention will be to slim down opportunities and recognize prospective culprits.
Then, test your theories systematically. Attempt to recreate the trouble in a managed setting. In the event you suspect a selected purpose or element, isolate it and validate if The difficulty persists. Just like a detective conducting interviews, inquire your code thoughts and Permit the outcomes guide you closer to the reality.
Fork out close attention to smaller specifics. Bugs often cover within the the very least anticipated locations—similar to a missing semicolon, an off-by-one mistake, or perhaps a race affliction. Be comprehensive and patient, resisting the urge to patch The problem with out thoroughly knowing it. Non permanent fixes could disguise the real challenge, only for it to resurface later on.
Last of all, preserve notes on Anything you experimented with and acquired. Just as detectives log their investigations, documenting your debugging system can preserve time for potential challenges and aid others realize your reasoning.
By imagining like a detective, developers can sharpen their analytical techniques, method challenges methodically, and become simpler at uncovering concealed issues in sophisticated devices.
Write Assessments
Crafting tests is one of the best tips on how to improve your debugging capabilities and In general development effectiveness. Assessments not merely support capture bugs early and also function a security Web that offers you confidence when creating modifications in your codebase. A properly-examined software is simpler to debug since it lets you pinpoint just the place and when a challenge happens.
Begin with unit exams, which give attention to personal features or modules. These tiny, isolated exams can rapidly reveal regardless of whether a particular piece of logic is working as envisioned. Every time a take a look at fails, you quickly know the place to search, substantially lowering the time used debugging. Device assessments are Specifically helpful for catching regression bugs—issues that reappear after Earlier currently being set.
Next, combine integration assessments and stop-to-end checks into your workflow. These support make certain that numerous aspects of your software function alongside one another efficiently. They’re specifically useful for catching bugs that come about in intricate methods with various parts or solutions interacting. If a little something breaks, your exams can show you which Section of the pipeline failed and underneath what circumstances.
Crafting exams also forces you to definitely Feel critically regarding your code. To test a aspect appropriately, you'll need to be aware of its inputs, expected outputs, and edge scenarios. This degree of knowledge Normally potential customers to better code framework and much less bugs.
When debugging a problem, producing a failing test that reproduces the bug might be a robust first step. When the exam fails constantly, you could concentrate on repairing the bug and check out your check move when The difficulty is resolved. This strategy makes sure that a similar bug doesn’t return Later on.
In a nutshell, crafting tests turns debugging from a annoying guessing activity right into a structured and predictable procedure—supporting you capture extra bugs, quicker and even more reliably.
Acquire Breaks
When debugging a tough problem, it’s straightforward to be immersed in the situation—gazing your screen for hours, attempting Remedy soon after Option. But One of the more underrated debugging tools is simply stepping away. Taking breaks helps you reset your mind, decrease aggravation, and often see the issue from a new perspective.
When you're too close to the code for too long, cognitive exhaustion sets in. You might start overlooking noticeable faults or misreading code that you choose to wrote just several hours before. In this particular condition, your brain gets to be much less efficient at trouble-resolving. A brief stroll, a coffee crack, or maybe switching to a unique activity for 10–quarter-hour can refresh your aim. Quite a few developers report discovering the root of a dilemma once they've taken time for you to disconnect, letting their subconscious do the job from the track record.
Breaks also assist prevent burnout, Primarily for the duration of for a longer time debugging sessions. Sitting down in front of a monitor, mentally caught, is not only unproductive but will also draining. Stepping away enables you to return with renewed Electrical power plus a clearer state of mind. You might quickly discover a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
When you’re stuck, a fantastic rule of thumb should be to set a timer—debug actively for forty five–60 minutes, then have a 5–10 moment break. Use that point to move all around, stretch, or do anything unrelated to code. It may come to feel counterintuitive, Particularly underneath tight deadlines, nonetheless it actually contributes to faster and simpler debugging Ultimately.
In brief, getting breaks is not a sign of weak point—it’s a sensible method. It offers your Mind Area to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight that often blocks your progress. Debugging can be a psychological puzzle, and rest is part of fixing it.
Study From Every Bug
Every single bug you come upon is more than just A brief setback—It truly is a possibility to grow like a developer. No matter if it’s a syntax mistake, a logic flaw, or a deep architectural concern, each can train you a thing important in the event you take some time to mirror and assess what went Erroneous.
Get started by inquiring you a couple of important queries as soon as the bug is fixed: What prompted it? Why did it go unnoticed? Could it have been caught earlier with better practices like unit tests, code reviews, or logging? The responses often expose blind places in the workflow or being familiar with and assist you Establish much better coding patterns going ahead.
Documenting bugs can be a fantastic routine. Preserve a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring challenges or popular faults—which you could proactively stay away from.
In group environments, sharing what you've acquired from the bug along with your peers is often Specially effective. Whether or not it’s via a Slack concept, a short generate-up, or a quick understanding-sharing session, encouraging Some others avoid the exact situation boosts group performance and cultivates a more powerful learning lifestyle.
A lot more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll commence appreciating them as critical areas of your development journey. In spite of everything, a few of the most effective developers are certainly not the ones who produce ideal code, but people that constantly study from their errors.
In the long run, each bug you correct provides a brand new layer on your skill set. So upcoming time you squash a bug, take a second to replicate—you’ll come away a smarter, far more able developer due to it.
Summary
Improving upon your debugging abilities normally takes time, observe, and patience — even so the payoff is large. It makes you a more productive, self-assured, and able developer. The next time you are knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become far better at That which you do.